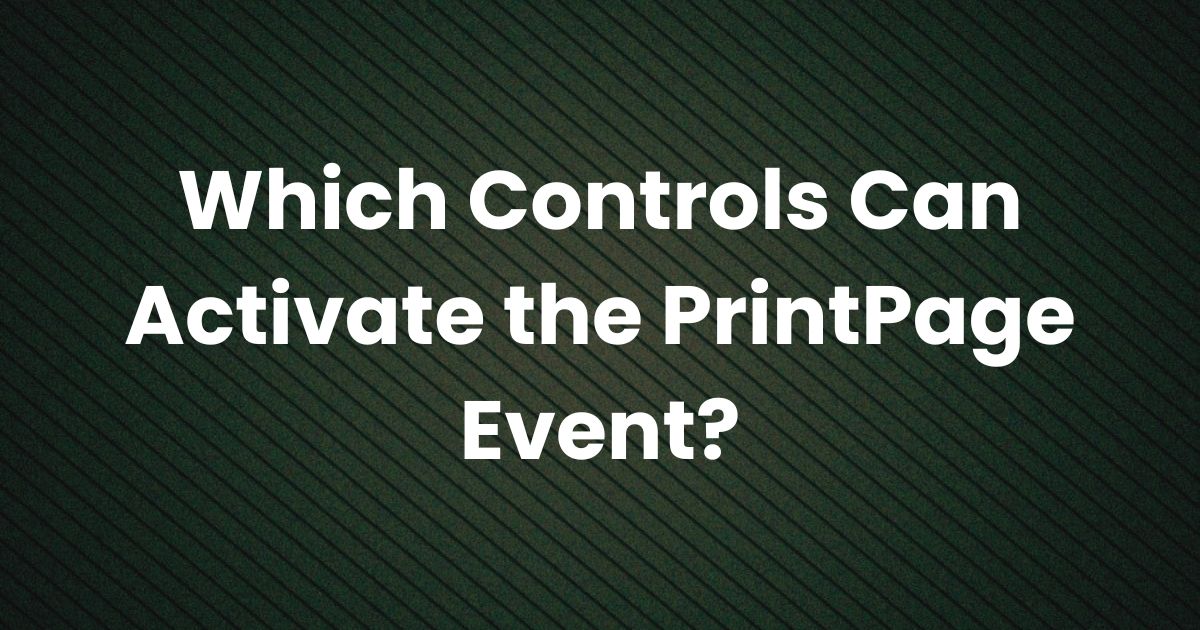
In many desktop applications, printing is an important feature that lets users produce physical copies of their work. In a .NET environment, the PrintPage event is key to controlling what gets printed and how it appears on the page. This article explains which controls can activate the PrintPage event and how they work together to trigger printing.
Introduction
When developing applications, you often need to provide users with the option to print documents, reports, or images. In the .NET framework, printing is managed by the PrintDocument control. The PrintDocument control has a PrintPage event that is raised each time a new page is ready to be printed. But which controls in your application can start or activate this event?
The short answer is that any control that calls the Print method on a PrintDocument object can activate the PrintPage event. Commonly, developers use buttons, menu items, or even keyboard shortcuts to trigger this process.
In this article, we will break down how the PrintPage event works, explain which controls typically initiate the printing process, and provide practical tips for integrating printing functionality into your application.
Understanding the PrintPage Event
Before diving into which controls can activate the event, it helps to understand what the PrintPage event is:
- PrintDocument Control:
The PrintDocument control in .NET manages printing tasks. When you call its Print method, the control begins the printing process. - PrintPage Event:
Each time a page is ready to be printed, the PrintPage event is raised. This event provides a graphics object that you use to draw text, images, or other content on the page. After the event handler finishes, the system sends the drawn content to the printer. - Event Handling:
You write an event handler for PrintPage to define what appears on the printed page. This includes specifying fonts, images, layout, and any other design elements.
Which Controls Can Activate the PrintPage Event?
The PrintPage event is triggered indirectly by calling the Print method on a PrintDocument object. The controls that can initiate this call are not limited by the PrintDocument itself; instead, they are the ones that handle user interaction in your application. Here are some common controls used to activate printing:
1. Button Controls
Button controls are one of the most common ways to start a print job. You can place a “Print” button on a form, and when a user clicks it, your code calls the Print method.
How It Works:
- The user clicks the Print button.
- The button’s click event handler calls the PrintDocument.Print() method.
- This call raises the PrintPage event, which your code handles to format the page for printing.
2. Menu Items
Menu items in a menu bar can also trigger printing. Applications often include a “File” menu with a “Print” option.
How It Works:
- The user selects the Print option from the menu.
- The menu item’s event handler calls the Print method on your PrintDocument control.
- This, in turn, fires the PrintPage event.
3. Toolbar Buttons
For applications that use toolbars, a toolbar button dedicated to printing can be an effective way to offer printing functionality.
How It Works:
- The user clicks the print icon on the toolbar.
- The event handler for this icon then invokes the PrintDocument.Print() method.
- The PrintPage event is triggered as a result.
4. Keyboard Shortcuts
Keyboard shortcuts (such as Ctrl+P) are also popular ways to initiate printing.
How It Works:
- The application listens for a specific key combination.
- When the user presses Ctrl+P, the shortcut event handler calls the Print method on the PrintDocument control.
- As with other controls, this starts the PrintPage event sequence.
5. Context Menu Items
In some cases, you might include a context menu that appears when the user right-clicks on a document or image. This menu can have a “Print” option as well.
How It Works:
- The user right-clicks on an object and selects “Print” from the context menu.
- The context menu item’s event handler calls PrintDocument.Print().
- The PrintPage event is raised to generate the printed output.
Practical Example: Using a Button to Activate Printing
Here’s a simple example in C# that demonstrates how a button can be used to activate the PrintPage event.
using System;
using System.Drawing;
using System.Drawing.Printing;
using System.Windows.Forms;
public class PrintForm : Form
{
private Button printButton;
private PrintDocument printDocument;
public PrintForm()
{
// Create a new button and set its properties
printButton = new Button();
printButton.Text = "Print";
printButton.Location = new Point(50, 50);
printButton.Click += new EventHandler(PrintButton_Click);
this.Controls.Add(printButton);
// Create a new PrintDocument and attach the PrintPage event handler
printDocument = new PrintDocument();
printDocument.PrintPage += new PrintPageEventHandler(PrintDocument_PrintPage);
}
private void PrintButton_Click(object sender, EventArgs e)
{
// Start the printing process when the button is clicked
printDocument.Print();
}
private void PrintDocument_PrintPage(object sender, PrintPageEventArgs e)
{
// Draw a simple string on the printed page
string printText = "Hello, world!";
Font printFont = new Font("Arial", 12);
e.Graphics.DrawString(printText, printFont, Brushes.Black, 100, 100);
}
[STAThread]
public static void Main()
{
Application.Run(new PrintForm());
}
}
Explanation:
- A button called
printButton
is added to the form. - When the user clicks the button, the
PrintButton_Click
event handler callsprintDocument.Print()
. - The PrintDocument control raises the PrintPage event, and the
PrintDocument_PrintPage
event handler executes to draw text on the printed page.
Tips for Implementing Printing in Your Application
Organize Your Code
Keep your printing logic separate from other application logic. This makes it easier to manage and update the PrintPage event handler without affecting other parts of your program.
Test Thoroughly
Printing can behave differently depending on the printer and the settings. Test your printing functionality with various printers and on different systems to ensure it works as expected.
Provide Feedback
Offer visual or textual feedback to the user when a print job is initiated. This can help prevent confusion if the print job takes time to start or if there are errors.
Handle Errors Gracefully
Include error handling in your print logic. If the printer is not available or an error occurs, display an informative message to the user.
Customize the Print Layout
The PrintPage event gives you full control over how the page is printed. Take advantage of this by customizing fonts, images, and layouts to match your application’s needs.
Conclusion
In summary, the PrintPage event in .NET is activated when the Print method of a PrintDocument control is called. While the PrintPage event itself is tied to the PrintDocument control, it is usually initiated by various user interface controls such as buttons, menu items, toolbar buttons, keyboard shortcuts, or context menu items.
By understanding which controls can trigger the PrintPage event and how to integrate them into your application, you can create a seamless and user-friendly printing experience. Whether you are developing a small desktop tool or a complex business application, the ability to print documents accurately and efficiently is a vital feature that enhances usability.
With the practical examples and tips provided in this guide, you now have a clear idea of how to set up and activate the PrintPage event in your application. Happy coding, and may your printed pages always come out just as you intend!
Also Check:
• Which Method Can Be Defined Only Once in a Program?
• Which Keyword Can Be Used for Coming Out of Recursion? An In-Depth Exploration
• Which Data Type Can an Array Not Hold?
• How Many Times Can HTML5 Events Be Fired? An In-Depth Exploration