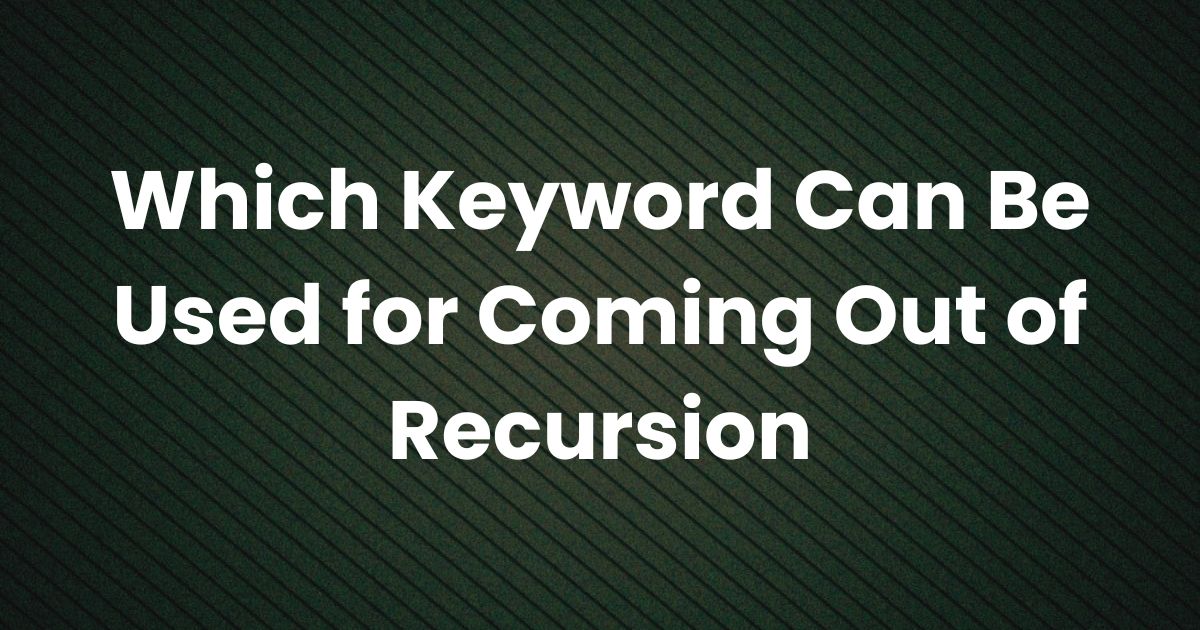
The straightforward solution is that the return
keyword is used to exit a recursive function call once a base case is met. In recursion, a function calls itself repeatedly until a condition—known as the base case—is satisfied. At that point, the function stops calling itself and uses return
to pass the final result back up through the chain of recursive calls.
Introduction
Recursion is a powerful programming technique where a function calls itself to solve a problem by breaking it down into smaller, more manageable sub-problems. While recursion can simplify the code and logic for certain tasks (like traversing a tree or computing a factorial), it must have a well-defined termination condition to prevent infinite recursion. This termination is achieved using a base case, where the function stops making further recursive calls.
The keyword that plays a central role in both ending the recursive calls and returning a value is return
. Without an appropriate return
statement, a recursive function would continue calling itself indefinitely, eventually leading to a stack overflow error.
Understanding Recursion
The Mechanics of Recursion
In a recursive function, there are two main components:
- Base Case:
This is the condition under which the function stops calling itself. Once the base case is reached, the function returns a specific value instead of making another recursive call. - Recursive Case:
This is the part of the function where the function calls itself with a modified argument, gradually working toward the base case.
Example: Factorial Function in Python
Consider a simple recursive function to compute the factorial of a number :
def factorial(n):
# Base case: if n is 0 or 1, return 1
if n == 0 or n == 1:
return 1
# Recursive case: n! = n * (n-1)!
else:
return n * factorial(n - 1)
# Example usage:
print(factorial(5)) # Output: 120
In this example:
- Base Case: When
n
is 0 or 1, the function returns 1. This is crucial to stop the recursion. - Recursive Case: For any other value of
n
, the function calls itself withn - 1
and multiplies the result byn
.
The return
keyword is used to output the result of each recursive call, effectively “coming out” of the recursion once the base case is reached.
The Role of return
in Exiting Recursion
Why return
Is Essential
- Termination of Recursive Calls:
Without a properreturn
statement in the base case, the recursive function would not know when to stop. Thereturn
keyword provides a clear exit from the recursion. - Passing Back Results:
The value returned by the base case is used by the previous recursive calls to build up the final result. For example, in the factorial function, thereturn 1
in the base case is multiplied by the subsequent values as the recursive calls unwind. - Control Flow:
return
effectively ends the execution of the current function call, allowing the program to move back to the previous call in the recursion stack.
What Happens Without return
If a recursive function lacks a proper return
in its base case, the function would either:
- Continue calling itself indefinitely, resulting in a stack overflow error.
- Return
None
(in languages like Python) for each call, which will not produce the intended result.
Common Misconceptions
Can Other Keywords End Recursion?
break
:
Thebreak
keyword is used to exit loops, not function calls. It cannot be used to terminate a recursive function.exit
orquit
:
These keywords or functions might terminate a program entirely, but they are not suitable for ending recursion gracefully within a function.
Proper Use of return
The key to proper recursive function design is ensuring that every branch of the recursion, especially the base case, includes a return
statement to deliver a result. This guarantees that each recursive call passes the correct value back to its caller, allowing the overall computation to complete successfully.
Conclusion
In conclusion, the return
keyword is essential for coming out of recursion. It is used to:
- Terminate recursive calls when a base case is reached.
- Pass the calculated result back through the chain of recursive calls.
- Ensure that the function does not continue indefinitely, which could lead to a stack overflow.
Understanding how to correctly implement the return
statement in a recursive function is crucial for writing efficient, reliable, and bug-free recursive algorithms.
Disclaimer: This article is intended for informational and educational purposes only. The concepts discussed are based on standard programming practices and may be implemented differently in various programming languages. Readers are encouraged to consult language-specific documentation and additional resources for more detailed guidance on recursion and the use of return
.
Also Check:
• Which Attribute Can Hold the JavaScript Version? An In-Depth Exploration
• Which of the Following Can Be Data in Pandas? An In-Depth Exploration
• Which Property of a Proton Can Change? An In-Depth Exploration
• How Many Times Can HTML5 Events Be Fired? An In-Depth Exploration
2 thoughts on “Which Keyword Can Be Used for Coming Out of Recursion? An In-Depth Exploration”