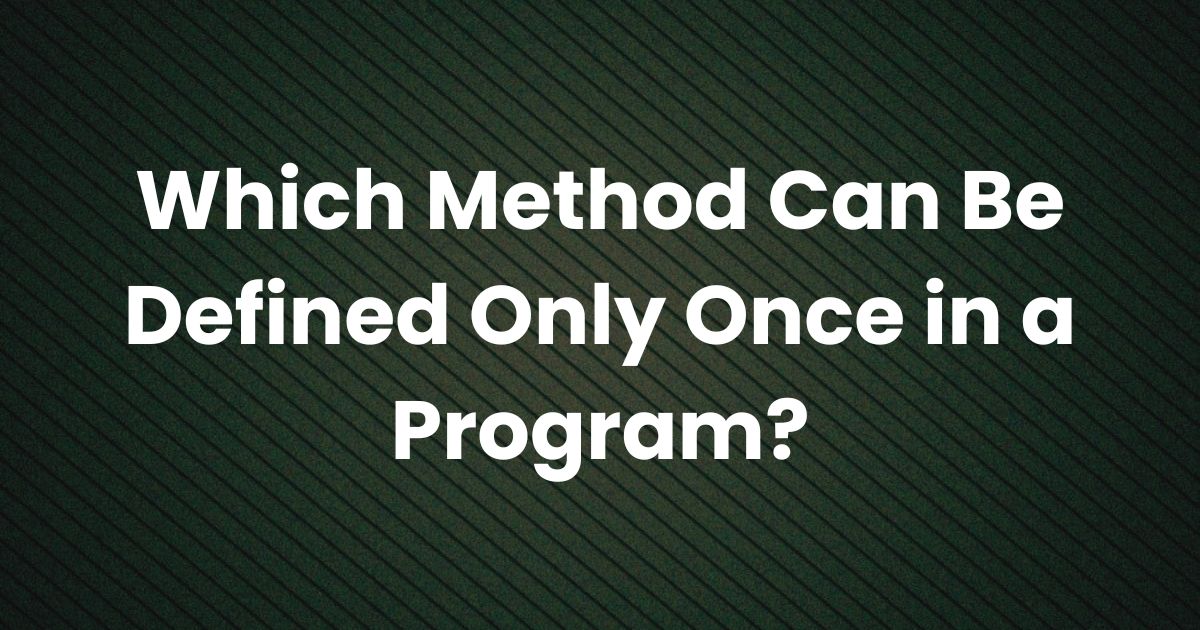
When you write a program, one of the most important parts is the entry point—the method where the program starts running. In many programming languages, this is called the main method. The main method can be defined only once in a program. This blog post explains why the main method is unique, what its role is, and why you can have only one per program.
Introduction
In programming, every application needs a starting point. This is the place where the computer begins executing your code. For most programming languages like C, C++, Java, and C#, this starting point is a method called main
. Because it serves as the entry point for the entire program, there can only be one main method. If there were more than one, the computer wouldn’t know which one to run first, leading to errors or unexpected behavior.
Understanding the importance of the main method is essential for both beginners and experienced programmers. This post breaks down the concept into simple language and offers a solution-based approach to understanding why the main method is defined only once.
What Is the Main Method?
The main method is a special function in many programming languages that tells the computer, “Start here!” It is the entry point of the program. Here are some key points:
- Starting Point: The main method is where the program begins execution.
- Single Instance: Since it is the designated starting point, you can define it only once in your program.
- Standard Signature: Different languages have specific ways to write the main method. For example:
- In Java, it looks like this:
public static void main(String[] args) { // Program execution starts here }
- In C or C++, it usually appears as:
int main() { // Program execution starts here return 0; }
- In C#, it might be:
static void Main(string[] args) { // Program execution starts here }
- In Java, it looks like this:
Why Can the Main Method Be Defined Only Once?
1. Clear Entry Point
The main method is the clear entry point of the program. When you run your program, the system looks for this one method to start execution. If there were multiple main methods, the system wouldn’t know which one to call, creating confusion.
2. Program Structure and Organization
Defining the main method only once helps maintain a clean and organized program structure. All the initial setup, such as initializing variables, configuring settings, or creating objects, is done in one place. This organization makes your code easier to read and debug.
3. Avoiding Conflicts
If multiple main methods existed, they could conflict with each other. Imagine trying to start a car with two ignition keys at the same time—it would lead to chaos. Similarly, multiple starting points in a program would result in errors and unpredictable behavior.
4. Consistency Across Platforms
Many programming languages and development environments are designed with the concept of a single entry point. This consistency helps programmers understand and work with different languages more easily. The rule that there is only one main method is part of a standard that keeps the coding experience smooth across various platforms.
How the Main Method Works in a Program
Program Initialization
When you launch your program, the operating system looks for the main method. It then begins executing the code inside this method line by line. Everything that your program does initially—like creating objects or calling other functions—starts from the main method.
Calling Other Methods
From the main method, you can call other methods and functions. These methods can be defined as many times as you want, but they all get executed from the single main method. This setup creates a clear flow of control in your program.
Ending the Program
When the main method finishes executing, the program ends. This is why it’s so important for the main method to be well-organized and handle any necessary cleanup before the program stops running.
Practical Tips for Using the Main Method
Keep It Simple
Try to keep the code inside your main method simple. Use it mainly for starting the program and calling other methods that handle more specific tasks. This makes your program easier to maintain.
Document Your Code
Since the main method is so crucial, add comments to explain what happens when the program starts. This documentation is helpful for anyone reading your code, including your future self.
Error Handling
Include proper error handling in the main method. If something goes wrong during initialization, your program should handle the error gracefully rather than crashing unexpectedly.
Testing
Test your main method thoroughly. Since it controls the flow of your entire program, any issues here can affect everything else. Use debugging tools to step through your code and ensure that the main method behaves as expected.
Conclusion
In summary, the main method is the unique entry point of a program. It is defined only once to provide a clear starting point for execution, maintain an organized program structure, avoid conflicts, and ensure consistency across different programming languages. Although you can define many other methods in your program, the main method remains singular, guiding the program from start to finish.
By keeping your main method simple, well-documented, and error-free, you create a strong foundation for your application. Whether you’re just starting out or have been coding for years, understanding the role of the main method is essential for building effective and reliable programs.
With this knowledge, you can now appreciate why the main method is the one method that can be defined only once in your program, ensuring that your code runs smoothly and predictably from the very first line.
Also Check:
• Which Keyword Can Be Used for Coming Out of Recursion? An In-Depth Exploration
• Can One Block of Except Statements Handle Multiple Exceptions
• Can the Return Type of the Main Function Be int
• Can a Call to an Overloaded Function Be Ambiguous? An In-Depth Explanation
1 thought on “Which Method Can Be Defined Only Once in a Program?”